- Details
- Written by: Stanko Milosev
- Category: Windows Forms
- Hits: 1224
using System; using System.Data; using System.Data.SqlClient; using System.Windows.Forms; namespace GridDataSetBindingSourceAndTableAdapterDynamicExample { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { string connectionString = "data source=localhost;initial catalog=TestCompleteTest;persist security info=True;Integrated Security=SSPI"; using (SqlConnection conn = new SqlConnection(connectionString)) { using (SqlCommand cmd = new SqlCommand("SELECT * FROM Names", conn)) { conn.Open(); DataSet ds = new DataSet(); SqlDataAdapter da = new SqlDataAdapter { SelectCommand = cmd }; da.Fill(ds); dataGridView1.DataSource = ds.Tables[0]; } } } } }Taken from here Example download from here
- Details
- Written by: Stanko Milosev
- Category: Windows Forms
- Hits: 1356

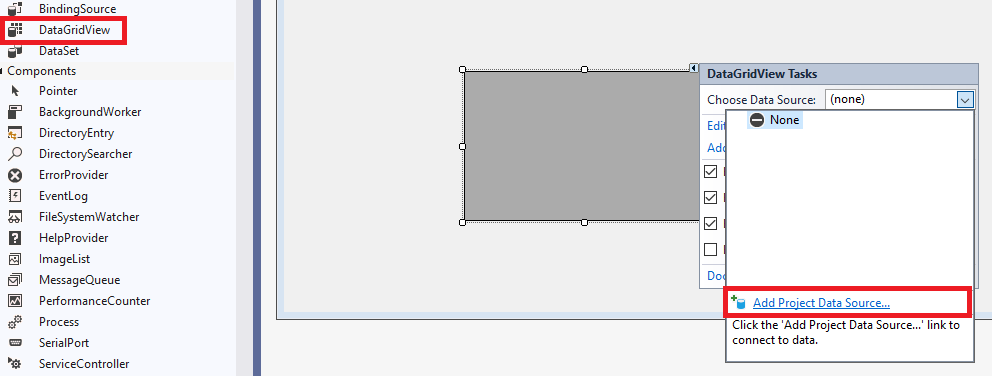
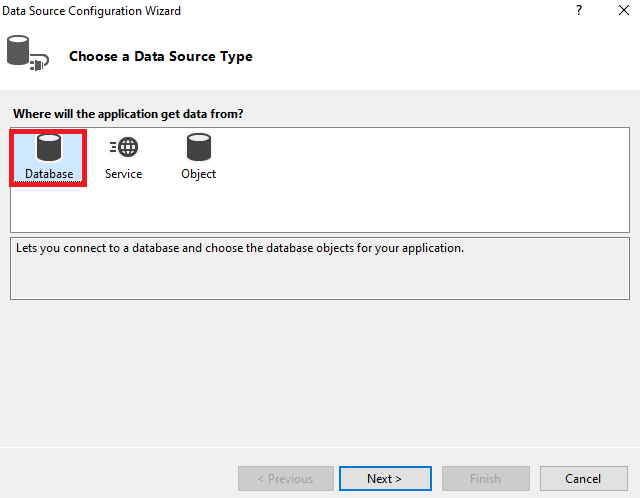
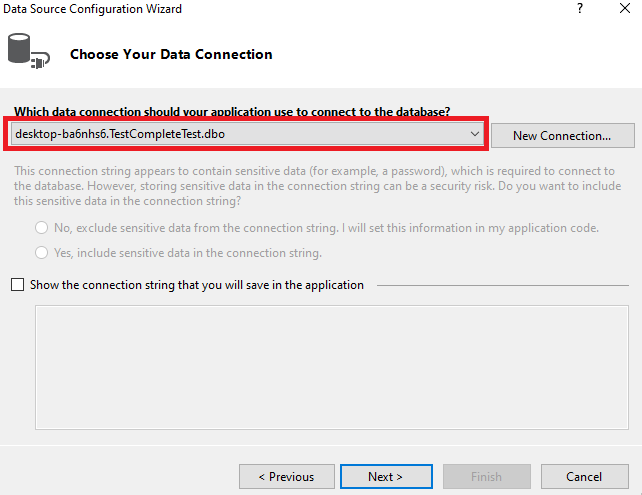

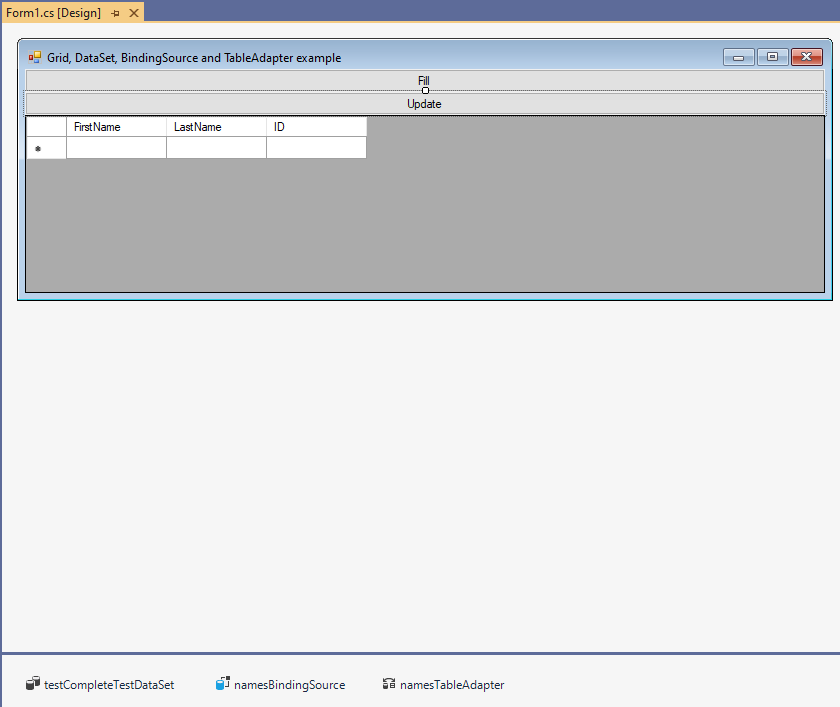
using System; using System.Windows.Forms; namespace GridDataSetBindingSourceAndTableAdapterExample { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { // TODO: This line of code loads data into the 'testCompleteTestDataSet.Names' table. You can move, or remove it, as needed. this.namesTableAdapter.Fill(this.testCompleteTestDataSet.Names); } private void Update_Click(object sender, EventArgs e) { this.namesTableAdapter.Update(this.testCompleteTestDataSet); } private void Fill_Click(object sender, EventArgs e) { this.namesTableAdapter.Fill(this.testCompleteTestDataSet.Names); } } }Here is SQL script to create table which I am using for this example:
CREATE TABLE [dbo].[Names]( [FirstName] [nvarchar](50) NULL, [LastName] [nvarchar](50) NULL, [ID] [int] NULL ) ON [PRIMARY]Example project in VS 2019 download from here
- Details
- Written by: Stanko Milosev
- Category: Windows Forms
- Hits: 1385
private void sdnButton1_Click(object sender, EventArgs e) { List<MyChild> stankosKids = new List<MyChild>(); stankosKids.Add(new MyChild { Name = "Velimir", Gender = "Male" }); stankosKids.Add(new MyChild { Name = "Hilda", Gender = "Female" }); List<MyChild> arnoldsKids = new List<MyChild>(); arnoldsKids.Add(new MyChild { Name = "Thomas", Gender = "Male" }); arnoldsKids.Add(new MyChild { Name = "Sabrina", Gender = "Female" }); List<MyChild> chucksKids = new List<MyChild>(); chucksKids.Add(new MyChild { Name = "Bruce", Gender = "Male" }); chucksKids.Add(new MyChild { Name = "Lee", Gender = "Female" }); List<MyParent> list = new List<MyParent>(); list.Add(new MyParent { ID = 1, FirstName = "Stanko", LastName = "Milosev", Address = "Herseler strasse 8", MyKids = stankosKids }); list.Add(new MyParent { ID = 2, FirstName = "Arnold", LastName = "Schwarzeneger", Address = "Whitehouse 1", MyKids = arnoldsKids }); list.Add(new MyParent { ID = 3, FirstName = "Chuck", LastName = "Norris", Address = "Las Vegas", MyKids = chucksKids }); ultraGrid1.SetDataBinding(list, null); } public class MyParent { public int ID { get; set; } public string FirstName { get; set; } public string LastName { get; set; } public string Address { get; set; } public List<MyChild> MyKids { get; set; } } public class MyChild { public string Name { get; set; } public string Gender { get; set; } }
- Details
- Written by: Stanko Milosev
- Category: Windows Forms
- Hits: 1770
private Image SetImageOpacity(Image image, float opacity) { try { //create a Bitmap the size of the image provided Bitmap bmp = new Bitmap(image.Width, image.Height); //create a graphics object from the image using (Graphics gfx = Graphics.FromImage(bmp)) { //create a color matrix object ColorMatrix matrix = new ColorMatrix(); //set the opacity matrix.Matrix33 = opacity; //create image attributes ImageAttributes attributes = new ImageAttributes(); //set the color(opacity) of the image attributes.SetColorMatrix(matrix, ColorMatrixFlag.Default, ColorAdjustType.Bitmap); //now draw the image gfx.DrawImage(image , new Rectangle(0 , 0 , bmp.Width , bmp.Height ) , 0 , 0 , image.Width , image.Height , GraphicsUnit.Pixel , attributes ); } return bmp; } catch (Exception ex) { MessageBox.Show(ex.Message); return null; } }My example how use this method:
try { float opacity = (float) trackBar1.Value / 100; panel1.BackgroundImageLayout = ImageLayout.Center; panel1.BackgroundImage = SetImageOpacity(myBitmap, opacity); } catch (Exception exception) { MessageBox.Show($@"Cannot to set opacity of picture. Error message: {exception.Message}"); }Notice that opacity is float and it can be between 0 to 1, everything bigger then 1 will have no impact. Example in Winforms you can download from here. Taken from here.